There are three main technologies that form the basis of most modern web sites: HTML, CSS and JavaScript. Whilst the inspector lets you play around with the HTML and CSS, the console is your playground for JavaScript. Switch to the Console tab. If there is any content in there already, clear it out by clicking the small trash can icon at the top left of the panel.
Compared with the inspector, the console may seem to be quite bare. That’s a good thing, as this is where errors in your web page will be displayed, so the less you see in here the better! The bulk of the console is taken up by the output section in the middle. Below it is a command line, into which you can directly type JavaScript code. Let’s give that a try by entering a simple arithmetic problem (that also happens to be valid JavaScript) into the command line, and pressing Enter to execute it:
1+1
The code you type will appear in the output section, marked by a chevron at the left. The result will be printed on the line below, marked with a left-facing arrow.
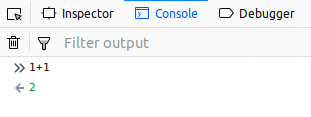
How about something a little more complicated?
(5*10)/2 + 3
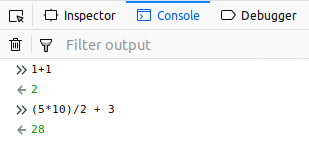
Try some other arithmetic problems. You can use the up and down arrow keys to step through your command history, so it’s easy to pick a previous command, edit it, then press Enter to run the new version.
Be careful of case JavaScript is a case-sensitive language. If you have a variable called a
, you can’t refer to it as A
— that would be a different variable entirely. When typing any of the JavaScript code below, take particular care to use exactly the same case as in the examples.
The command line can even handle multi-line input. You can force a line break by pressing Shift-Enter, but it will also switch to multi-line mode automatically if it detects that you’ve opened a block of code and haven’t closed it. Type in the following to create a new function, pressing Enter after each line:
function square(x) {
return x*x;
}
The console will show a result of undefined
. That’s nothing to worry about, it’s just the default value that JavaScript uses when an instruction doesn’t return anything more useful. Now that we’ve created a function, we can use it to calculate the square of a number:
square(256)
65536
The console also integrates with the other developer tools. This can be particularly useful when working with the inspector. Whatever is selected in the DOM list will automatically be assigned to a special variable called $0
. Right-click on the page heading and select Inspect Element again, then switch back to the console. Type $0
into the command line then press Enter, and you should see this:
See the little triangle to the left of the result? You can click that to expand the output, showing all the JavaScript properties that are attached to the DOM element. Scroll down to the textContent
property near the bottom, for example, and you’ll see the string that appears as the header on the page. As well as viewing these properties, you can also change many of them using JavaScript. Type this into the command line to alter the heading once again:
$0.textContent = "Do more with JavaScript!"
Type $0
again, and this time move your mouse over the little square target icon to the right of the output. The <h1>
is highlighted in the page, just as it was when you moved your mouse over the DOM entry in the inspector. Click on the icon and you’ll be taken to the inspector and the element in question will be highlighted. This feature can be invaluable when the console contains multiple elements. Here’s one way to find all the links in the page:
document.querySelectorAll("a")
This time the output is a “NodeList” — a structure that is similar to an array, which contains DOM elements. The list will be truncated in the console, but you can click on the triangle at the left if you want to view all the entries. Click on the square target button for any of them and you’ll be taken to the inspector, with the link highlighted. Return to the console and you’ll find that $0
is no longer your <h1>
element, but is now the link. Type $0.href
and the console will print the URL that the link points to.
Interactive use of the console is useful, but the browser also exposes some console functions that can be called from within your own code. By far the most commonly used of these is console.log()
, which is used to write to the console, even when the developer tools are closed.
In the early days of web development it was common to use the alert()
function to output debugging data to a dialog on screen. But alert()
could only work with a single argument at a time, and anything you did pass would be converted to a string. Type these two lines into the command line to see how useless alert()
is when passed a complex data type, such as a JavaScript object (a variable type that stores data in name:value pairs):
alert("Fred Flintstone")
alert({firstName: "Fred", lastName: "Flintstone"})
Not terribly useful, right? Now let’s try that second example using console.log()
instead:
console.log({firstName: "Fred", lastName: "Flintstone"})
Not only is the output far more useful, but there’s no dialog to dismiss, and the execution of your program doesn’t stop while it’s waiting for you to click the OK button. As a last example, let’s combine some of the commands we’ve used previously into one single console.log()
:
console.log("On this page there are ", document.querySelectorAll("a").length, " links. The currently selected element in the DOM tree is: ", $0)
In real code you would usually split your console.log()
calls into separate, shorter messages. But the ability to log out several different parameters just by comma-separating them, provides a huge amount of flexibility.